CS 2120: Class #2¶
Welcome back¶
Let’s get started right away...
Quick Activity
What did you do this weekend/week?
What did you do with Python this weekend/week?
Heads up: Before we get to the “super awesome fun stuff”, we’ve got to cover the basics. I understand that the basics aren’t super awesome. Don’t worry, we’ll get there. But we can’t get there without the basics.
- For motivational purposes, here’s what Google Image Search gave me when I searched for “awesome robot”:
- At this point I would like to remind the class that I did NOT create a lot of this material -_-
- I’m sooooo sorry about this
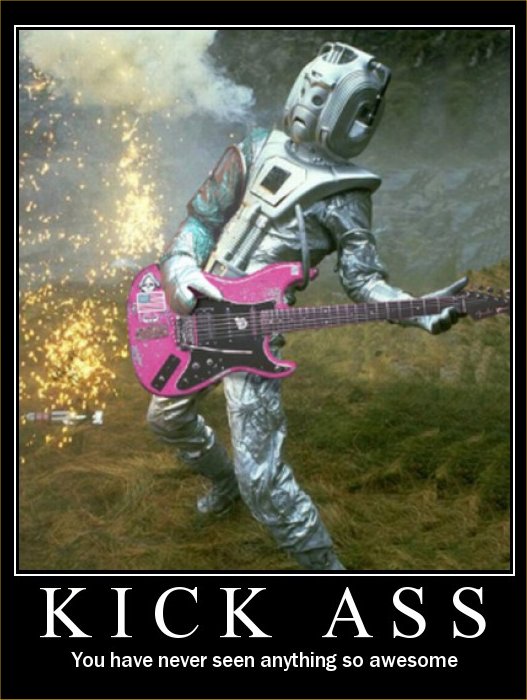
What’s a program?¶
- The stuff in the computers
- A thing that does stuff
- A recipe
- A sequence of instructions that specifies exactly how to perform a computation
Activity
Explain to a partner how you would go about making breakfast in the morning.
Activity
Someone explain to me how to make breakfast in the morning.
- There, that’s basically a program.
What’s debugging?¶
- Mystery novel
- A logic puzzle
- How you fix your mistakes
- If you’re an experimental scientist, it’s a lot like “protocol optimization”
Quick Activity
Have you seen any Python errors yet?
What were they?
Did you understand them?
Languages¶
- What’s the difference between a formal, and a natural, language?
- Why is ambiguity so important to natural language?
- Why is ambiguity deadly for a formal language?
Activity
Do you think there is a limit to what I can describe with a formal language?
Can I describe anything? Any computation?
HINT: Is the following statement true or false: “This statement is false.”
- The world is a screwed up, scary, place (for mathematicians, anyways). If you want to fall down this particular rabbit hole:
Okay, we’re done with the background, let’s get on with the real stuff¶
Activity
Write a (single-line) Python program that prints a witty message, of your choice, to the console.
Values (not the family kind)¶
- Values are things that a program manipulates.
- Strings: “abcdef”
- Integers: 7, 42, 97
- Floating-point numbers: 3.792, 0.000000000005
Notice how I described the type of each value along with the value itself
Computers are exceptionally stupid. You must be completely explict about everything.
To a computer, the integer 1 is not necessarily the same thing as the floating point number 1.0... because they have different types
Many of the errors you will make in programming result from mixing types inappropriately.
Some languages (e.g., C, Fortran, Java) are very militant about types. You have to be totally explicit about them.
Python is a little more relaxed. You can be explicit, but you don’t have to be. Python will guess if you don’t tell it.
Upside: less to worry about and less clutter in your code.
Downside: a longer rope gives you more fun and exciting ways to hang yourself!
- Can I ask Python to tell me its guess for the type of a value?
>>> type(12) <type 'int'> >>> type('Witty remark') <type 'str'> >>> type(3.75) <type 'float'>
- It’s kinda’ easy to tell the type of a value isn’t it?
- Most of the time.
Activity
Give a partner a value and have them tell you the type. Pleas ask if you run into a problem here.
Variables¶
Probably the most important feature of a procedural programming language.
If you’re going to pay attention only once this term... now’s the time.
Variables let you store values in a labelled (named) location
- You store values into variables by using the assignment operator =
>>> a=5 >>> m='Variables are fun'
For historical reasons, we’re stuck with the ‘=’ symbol for assignment, but it doesn’t really mean the same thing as the ‘=’ sign in math.
In math when we write ‘a = 5’ we mean that ‘5’ and ‘a’ are equivalent as they exist. We’re not asking to change anything; we’re making a statement of fact.
- In Python when we write
>>> a=5
... we’re saying “Hey, Python interpreter! Create a variable named
a
and store the value5
in it. This isn’t a statement of fact, it’s an order!
What can you do with variables?¶
Anything you can do with values.
- For example, we can add variables:
>>> a = 5 >>> b = 7 >>> a+b 12 >>> b=5 >>> a+b 10
This seems pretty lame and straightforward now, but it’s this ability to store results that will let us do all the cool stuff later.
Activity
- Assign various values of types string, integer and float to variables.
- Try adding variables of the same type. What happens?
- Try adding variables of different types. What happens?
- Try the assignment 5=a. What happens?
- Figure out how to display the current contents of a variable.
Choosing variable names¶
- You can use whatever you want, within a few restrictions set by the language.
- Python wants variable names that begin with a letter of the alphabet and limits what non-alphanumeric characters you can use
- A good choice is a variable name that is descriptive of what the variable is meant to contain.
- good:
density
- less good:
d
- bad:
definitely_not_density
- good:
Activity
Suppose you’re a big fan of ‘80s Arena Rock. Create two variables, named def
and leppard
, set them to 19
and 87
respectively, then add them.
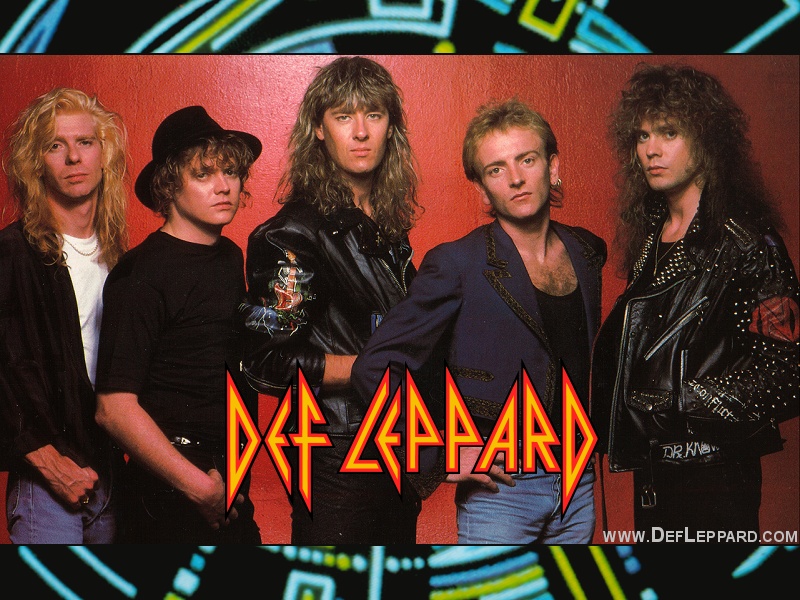
- What happened? (To your code, not the band!)
Statements¶
A statement is an order to Python: “do something”.
An instruction that can be executed by the interpreter.
You type in the statement, press Enter, and Python does what you asked (or at least tries to).
Some statements produce immediate output, some just change things ‘behind the scenes’.
We’ve already been using assignment statements (
=
), but there are lots of other kinds of statements.- e.g., you should already have discovered the
print
statement: >>> leppard = 87 >>> print leppard 87
- e.g., you should already have discovered the
Expressions¶
An expression is, roughly, a thing that can be crunched down to a value.
- More precisely, an expression is a combination of:
values (e.g.,
5
)variables (e.g.,
leppard
)- operators (e.g.,
+
) >>> leppard * 2 + 7 181
- operators (e.g.,
Operators¶
- Operators are symbols that tell Python to perform computations on expressions.
- e.g., +, -, *, /
Activity
Generate expressions to:
- Add two variables
- Multiply two variables
- Add a third variable to 2
- Divide 3 by 1
ARE YOU READY FOR THIS?
- Convert a temperature in Celsius to Fahrenheit.
-
Order of Operations¶
Python doesn’t blindly evaluate expressions; it follows the usual order of operations you learned in public school math class.
- If you want things done in some other order, you can use
()
to make it explicit: >>> 2 + 5 * 2 12 >>> (2 + 5) * 2 14 >>>
- If you want things done in some other order, you can use
Are operators just for numbers?¶
- Nope! Values of all sorts have operators that work on ‘em.
Activity
- Experiment with the operators you know on strings (instead of just integers).
- Which ones work? What do they do?
- Try mixing strings and integers with various operators. What happens there?
Doing sequences of things¶
So far we’ve just been entering one line at a time into the Python interpreter.
That’s not going to scale very well for most of the stuff we want to do...
You can store an (arbitrarily long) series of statements in a file, and then ask Python to run that file for you.
The Python interpreter will execute each line of the file, in order, as if you’d typed them in.
- There are lots of ways to run scripts. Suppose you put a series of statements into a file called
myprogram.py
- from the shell:
$ python myprogram.py
oripython myprogram.py
- from the interpreter:
>>> execfile('myprogram.py')
- if you’re using Ipython:
%run myprogram
- from the shell:
- There are lots of ways to run scripts. Suppose you put a series of statements into a file called
- To edit the script, you can use any text editor that you want. You’ll have an easier time with one that is “Python aware”, though.
- Wut?
- EPD has the IDLE editor/IDE built in. It’s not bad.
- PythonAnywhere has their own browser-based editor.
- If you installed your own Python, you probably already have your own favourite editor.
Activity
Consider the sentence Def Leppard is a poor substitute for Van Halen
. Write a program that stores each word of that sentence
in it’s own variable, and then prints the whole sentence to the screen, using only a single print statement.
For next class¶
- Read the rest of chapter 2 of the text
- Read chapter 3 of the text